keep on programming –> make a living –> marry a girl –> raise a child –> teach him to program !~
Web Hotpot. Source code can be found in our github.
We always see a lot of cool websites. Also, we heared about website elements, such as servers: wamp, lighttpd, frameworks: web.py, twisted, javascripts: angularJS, BootStrap, and some other website techniques. This time, My friend Zhiyue Zu and I want to build our own website systematically, from front to end, from interface to database. We are willing to share our knowledge with others. Also, we’ll appreciate that someone tell us amazing techniques or frameworks.
Ths website is a simply a navigation website, we call it WebTech Navigate. We desire to collect information about techniques that will be use when building a website.
vim
A lot of codeing is necessary to build a website, so editor is important. I heared about emacs, but I’m still more customed in vim.
vim is a powerful editor because of brunches of plugins. Personally, I recommand vundle rather than pathogen because vundle is more advanced to manage the plugins. Excellent plugins can be found in VimAwesome. I have a .vim configuration in my github. So each time when I have to config a new linux machine, I’ll:
1 check if vim is well installed. If not, reinstall.
2 use scp, winscp or git to copy the .vim file.
3 Install vundle
4 Launch vim and run
nginx
I used lighttpd to build my snake game. This time, we choose nginx which seems more professional. Nginx can provide basic HTTP or SMTP service. With fastcgi module, nginx can support all kinds of script files. But when nginx cooperate with nodejs which already has a server service, we will use nginx as a reverse proxy to control the load balance.
1 install nginx in Ubuntu
2 start the server
3 control the server. signals can be stop, quit, reload and reopen
4 revise the configuration file, at /etc/nginx/sites-enabled/
As described in Using nginx as HTTP load balancer There are 3 ways provided by Nginx.
- round-bin: requests to the application servers are distributed in a round-robin fashion
- least-connected: next request is assigned to the server with the least number of active connections
- ip-hashed: a hash-function is used to determine what server should be selected for the next request (based on the client’s IP address)
Load balance across multiple application instances is a commonly used technique for optimizing resource utilization, maximizing throughput, reducing latency, and ensuring fault-tolerant configurations.
Reverse proxy implementation in nginx includes in-band (or passive) server health checks. If the response from a particular server fails with an error, nginx will mark this server as failed, and will try to avoid selecting this server for subsequent inbound requests for a while.
Make sure nginx reload the configuration file, a simple reverse proxy is set up. It works!
node.js
node.js is an event-driven I/O server-side JS environment. It is brillant because with this we can use JS in frot, back and even the server. Node.js can solve the 10k problem easily.
Use nvm to install, manage or transfer node.js for different versions. Use npm to manage javascript packages.
Node provide a bunch of APIs, which can be seen in API Documentation.
Here just list some of them:
- Console
- HTTP
- HTTPS
- fs(File System)
- Path
- Modules
syntax for using modules:
For example:
Another important thing is module loading system.
Built in modules are core modules, use:
user’s modules can be used in the way:
There are two ways to export:
1 Add functions and objects to the root of your module, you can add them to the special exports object.circle.js
foo.js
:
2 If you want the root of your module’s export to be a function (such as a constructor) or if you want to export a complete object in one assignment instead of building it one property at a time, assign it to module.exports instead of exports
square module is defined in square.js
:
bar.js
:
Way 1 is recommanded, so that it’s scalable.
###nvm
The usage of nvm can be found at Node Version Manager.
Install nvm with script:
or with Wget:
nvm repositry can be found at ~/.nvm
Install/use/run a certain version of node.js:
or to use the stable version
Find the path of a certain version of node.js:
set default version of node.js:
use ls to check all the version installed, and the sversion of stable and default.
npm
npm is package manager for javascript, here we use it as the manager for node.js.
Search on npm website to find the package you need, and use the command to install the package:
Moreover, it’s better to use the package.json. Inside the project, run:
If it’s a new project download from other place, already have the json file, just run the command, so the npm will install the dependencies automatically:
When install some package, which contain some global commands, such as express generator, run:
When installing a package, which need to be record in the json, run:
Update:
Uninstall:
Uninstall along with the json record:
express
Express is a web framework for node.js.
Install express inside a project:
Or, we want to create a project based on node.js and express, we can use express generator directlly, like described in the Express application generator:
Express has lots of APIs too. Express API 4.x.
application
|
|
There are several general functions:
router
example of basic route:
req and res can be treated as a data set or structure, they have many useful members
like req.ip req.hostname req.cookies req.secure.
mongoose
grunt
Javascript Tsak runner.
Grunt should be installed as global:
There are many grunt plugins which can support the js tasks. Use the way to install them and add into package.json
in the developing environment.
Inside the folder, there would be a file named as Gruntfile.js
or Gruntfile.coffee
.
Example of Grunt file:
options:
file compact:
Grunt can be used to:
1 copy the min.js in developing environment to ./public/javascript
as same as css files.
2 compress your own js, css file into destination
3 watch the js files to see if there is syntax error.
Useful tasts:
- concat
grunt-contrib-concat. Concatenating files with a custom separator - jshint
grunt-contrib-jshint. Valid files with JSHint - uglify js
grunt-contrib-uglify. Minify js files. - minify css
grunt-contrib-cssmin. Minify css files. - watch
- copy
mongodb
Front end
HTML
markup language. HTML is used to establish a page’s structure. It also lets us add text, links and images.
body contains the actual content of the web page.
general usage:
headings. h1 to h6. <h1>text</h1>
paragraph. <p>text</p>
Links. <a href="">text</a>
Images. <img src=" ">
Lists.
CSS
CSS is used to control the design and layout of the page.
use the syntax to load css to a html:
####rules
Start with selector, inside the brace, are property and value pairs.
|
|
|
|
####colors
- Word: red
- RGB: 0 to 255. rgb(102,153,0)
- Hex: 00 to ff. #9933CC
general
font-family
font-size (pixels, ems, or rems),px, em
background-color
background-image
layout
padding; border; margin; position
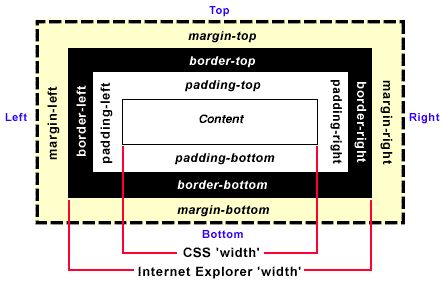
####selector
CSS selector
jQuery
jQuery is a Javascript Library. write less, do more
For a comparison, STL(standard temlate Library) in C++, which provide convenient functions by encapusulationg the lower leverl of C++.
We can know jQuery API fron official website. Also can learn from codeacademy and w3schools.
jQuery library contains the following features:
- HTML/DOM manipulation
- CSS manipulation
- HTML event methods
- Effects and animations
- AJAX
- Utilities
####Syntax:
Like the css selector:
The document ready event:
Event
- Mouse
click; dbclick; mouseenter; mouseleave; - Keyboard
keypress; keydown; keyup; - Form
submit; change; focus; blur; - Document/Window
load; resize; scroll; unload;
pass a function to the event:
|
|
Effect
Hide/Show; Fade; Slide; Animate; … See the syntax on the API.
Manipulate HTML/CSS
Get content
Set content
Add or remove
change the css attrbite
…
jQuery AJAX
AJAX = Asynchronous JavaScript and XML.
AJAX is about loading data in the background and display it on the webpage, without reloading the whole page
The load() method loads data from a server and puts the returned data into the selected element:
eg:
The jQuery get() and post() methods are used to request data from the server with an HTTP GET or POST request:
But generally, modern framework like AngularJS, react, they have their own mechanism to update certain DOM.
AngularJS
sementicUI
domain name
AWS EC2
Extra
###Disqus
###Google analysic